Please scroll down for latest Programs 👇
Week-11 Program-01
Due on 2024-04-11, 23:59 IST
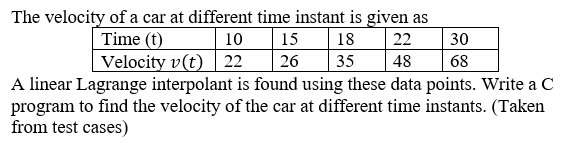
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 25 | The respective value of the variable v is: 56.42 | The respective value of the variable v is: 56.42 | Passed |
Test Case 2 | 16 | The respective value of the variable v is: 28.74 | The respective value of the variable v is: 28.74 | Passed |
Week-11 Program-02
Due on 2024-04-11, 23:59 IST

Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 0
1 | The integral is: 0.335000 | The integral is: 0.335000 | Passed |
Test Case 2 | 1
3 | The integral is: 8.680000 | The integral is: 8.680000 | Passed |
Week-11 Program-03
Due on 2024-04-11, 23:59 IST
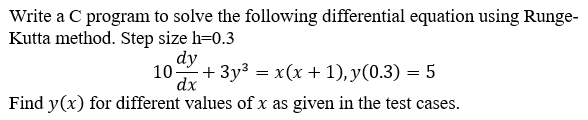
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 0.9 | y=1.777165 | y=1.777165 | Passed |
Test Case 2 | 1.2 | y=1.468128 | y=1.468128 | Passed |
Week-11 Program-04
Due on 2024-04-11, 23:59 IST
Write a C program to check whether the given input number is Prime number or not using recursion. So, the input is an integer and output should print whether the integer is prime or not. Note that you have to use recursion.
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 13 | 13 is a prime number | 13 is a prime number\n
| Passed after ignoring Presentation Error |
Test Case 2 | 40 | 40 is not a prime number | 40 is not a prime number\n
| Passed after ignoring Presentation Error |
Week-12: Program-01
Due on 2024-04-18, 23:59 IST
Write a program in C to find the factorial of a given number using pointers.
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 5 | The Factorial of 5 is : 120 | The Factorial of 5 is : 120\n
| Passed after ignoring Presentation Error |
Test Case 2 | 15 | The Factorial of 15 is : 1307674368000 | The Factorial of 15 is : 1307674368000\n
| Passed after ignoring Presentation Error |
Week-12: Program-02
Due on 2024-04-18, 23:59 IST
Write a C program to print the Record of the Student Merit wise. Here a structure variable is defined which contains student rollno, name and score.
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 3
1
Santanu
700
2
Aparna
550
3
Vivek
900 | The Merit List is :\n
3 Vivek 900\n
1 Santanu 700\n
2 Aparna 550 | The Merit List is :\n
3 Vivek 900\n
1 Santanu 700\n
2 Aparna 550\n
| Passed after ignoring Presentation Error |
Week-12: Program-03
Due on 2024-04-18, 23:59 IST
Write a C program to store n elements using Dynamic Memory Allocation - calloc() and find the Largest element
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 5
200
300
500
100
400 | Largest element = 500.00 | Largest element = 500.00 | Passed |
Week-12: Program-04
Due on 2024-04-18, 23:59 IST
Write a C program to add two distance given as input in feet and inches.
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 40
11
10
11 | Sum of distances = 51 feet 10 inches | Sum of distances = 51 feet 10 inches | Passed |
Test Case 2 | 10
5
20
5 | Sum of distances = 30 feet 10 inches | Sum of distances = 30 feet 10 inches | Passed |
No comments:
Post a Comment
Keep your comments reader friendly. Be civil and respectful. No self-promotion or spam. Stick to the topic. Questions welcome.